最近有一个检测网站是否被墙的需求,找了几个网站,发现IT狗的API比较方便。
API详情
我们先看看IT狗的API的调用方式:
获取页面
1 2 3 4
| URL:https://www.itdog.cn/firewall/[domain] 请求方式:POST 请求主体:more=no&button_click=yes 返回:text/html
|
此处的[domain]
即要检测的域名(一级域名),POST请求的主体无需变动。
这个API会返回一段HTML,即在浏览器打开时的页面。
防火墙检测
1 2 3 4
| URL:https://www.itdog.cn/public/ajax.aspx?type=firewall 请求方式:POST 请求主体:host=[domain]&token=[token] 返回:text/html(但是格式为JSON)
|
此处的[domain]
即要检测的域名(一级域名),[token]
的获取稍后会提到。
若请求成功,其返回内容如下:
1 2 3 4 5
| { "type":"success", "host":"pai233.top", "firewall":false }
|
请求失败/请求过快则返回空。
DNS检测
1 2 3 4
| URL:https://www.itdog.cn/public/ajax.aspx?type=dns_error 请求方式:POST 请求主体:host=[domain]&token=[token] 返回:text/html(但是格式为JSON)
|
此处的请求主体与防火墙检测的一样,但返回内容稍微有点不同。
若请求成功,其返回内容如下:
1 2 3 4
| { "type":"success", "dns_error":false }
|
同样的,请求失败/请求过快返回空。
获取Token
要调用IT狗的API,首先要获取到请求Token。那这个Token去哪里找呢?在网页端测试的页面中(即第一个获取页面API),我们可以发现到这一段:
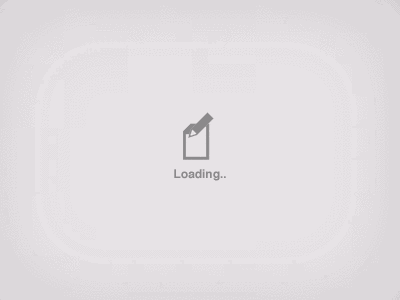
这个就是我们需要的Token了。所以我们可以写出以下代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| let _ = require("axios").default const cookie = require("tough-cookie"); const cookieSupporter = require("axios-cookiejar-support").default const JAR = new cookie.CookieJar() const https = require("https") let domain = "pai233.top" _ = cookieSupporter(_) _.defaults.jar = JAR _.defaults.withCredentials = true let html =await _.post('https://www.itdog.cn/firewall/'+domain,"more=no&button_click=yes",{ headers: { 'Referer': 'https://www.itdog.cn/firewall/' } }); let tokenReg = new RegExp(/var token='(.*)'/g) let token = tokenReg.exec(html.data)[1]
|
此时的token
变量里,已经成功存储了我们需要的Token。
发起请求
既然已经获取到了Token,那么请求就简单了:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| let _ = require("axios").default const cookie = require("tough-cookie"); const cookieSupporter = require("axios-cookiejar-support").default const JAR = new cookie.CookieJar() const https = require("https") let domain = "pai233.top" _ = cookieSupporter(_) _.defaults.jar = JAR _.defaults.withCredentials = true let html =await _.post('https://www.itdog.cn/firewall/'+domain,"more=no&button_click=yes",{ headers: { 'Referer': 'https://www.itdog.cn/firewall/' } }); let tokenReg = new RegExp(/var token='(.*)'/g) let token = tokenReg.exec(html.data)[1] let gfwStatus = await _.post('https://www.itdog.cn/public/ajax.aspx?type=firewall',"host="+domain+"&token="+token,{ headers: { 'Referer': 'https://www.itdog.cn/firewall/'+domain } }) let dnsStatus = await _.post('https://www.itdog.cn/public/ajax.aspx?type=dns_error',"host="+domain+"&token="+token,{ headers: { 'Referer': 'https://www.itdog.cn/firewall/'+domain } }) console.log(gfwStatus,dnsStatus)
|
这样就实现了检测域名是否被墙的需求。